
You can use GDB’s codedisassemble command to disassemble individual functions. The objdump (80x86) or i386-elf-objdump (SPARC) utility can disassemble entire user programs or object files. Until you have implemented syscalls sufficiently, you can use hex_dump to check your argument passing implementation (see Startup). The default syscall handler just handles exit(). Notably, printf invokes the write syscall. Every reasonable program tries to make at least one syscall ( exit) and most programs make more than that. You’ll have to implement syscall before you see anything else. All my user programs die upon making a syscall. If the stack isn’t properly set up, this causes a page fault. The basic C library for user programs tries to read argc and argv off the stack. This will happen if you haven’t implemented argument passing (or haven’t done so correctly). All my user programs die with page faults. See the comment underneath the kernel stack diagram in threads/thread.h about the importance of keeping your struct thread small. It’s also possible that you’ve made your struct thread too large.
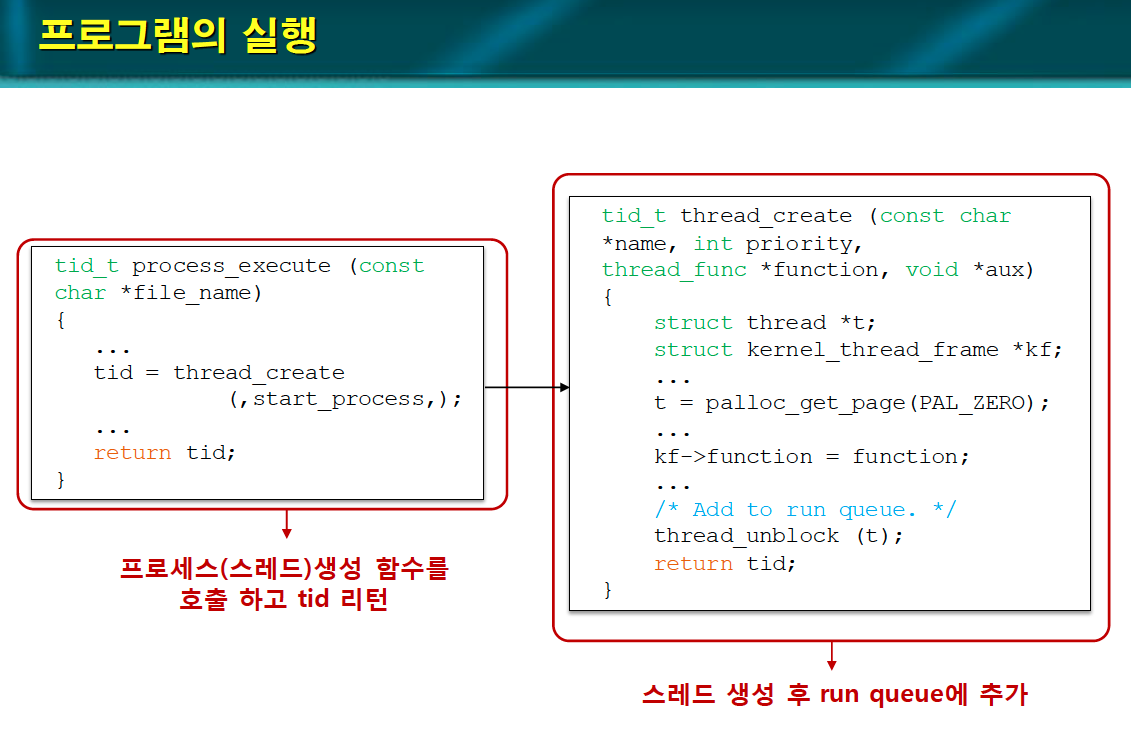
If you’re allocating large structures or buffers on the stack, try moving them to static memory or the heap instead. This happens when you overflow your kernel stack. The kernel always panics with assertion is_thread(t) failed. Is the file system full? Does the file system already contain 16 files? The base Pintos file system has a 16-file limit. Is your file name too long? The file system limits file names to 14 characters.
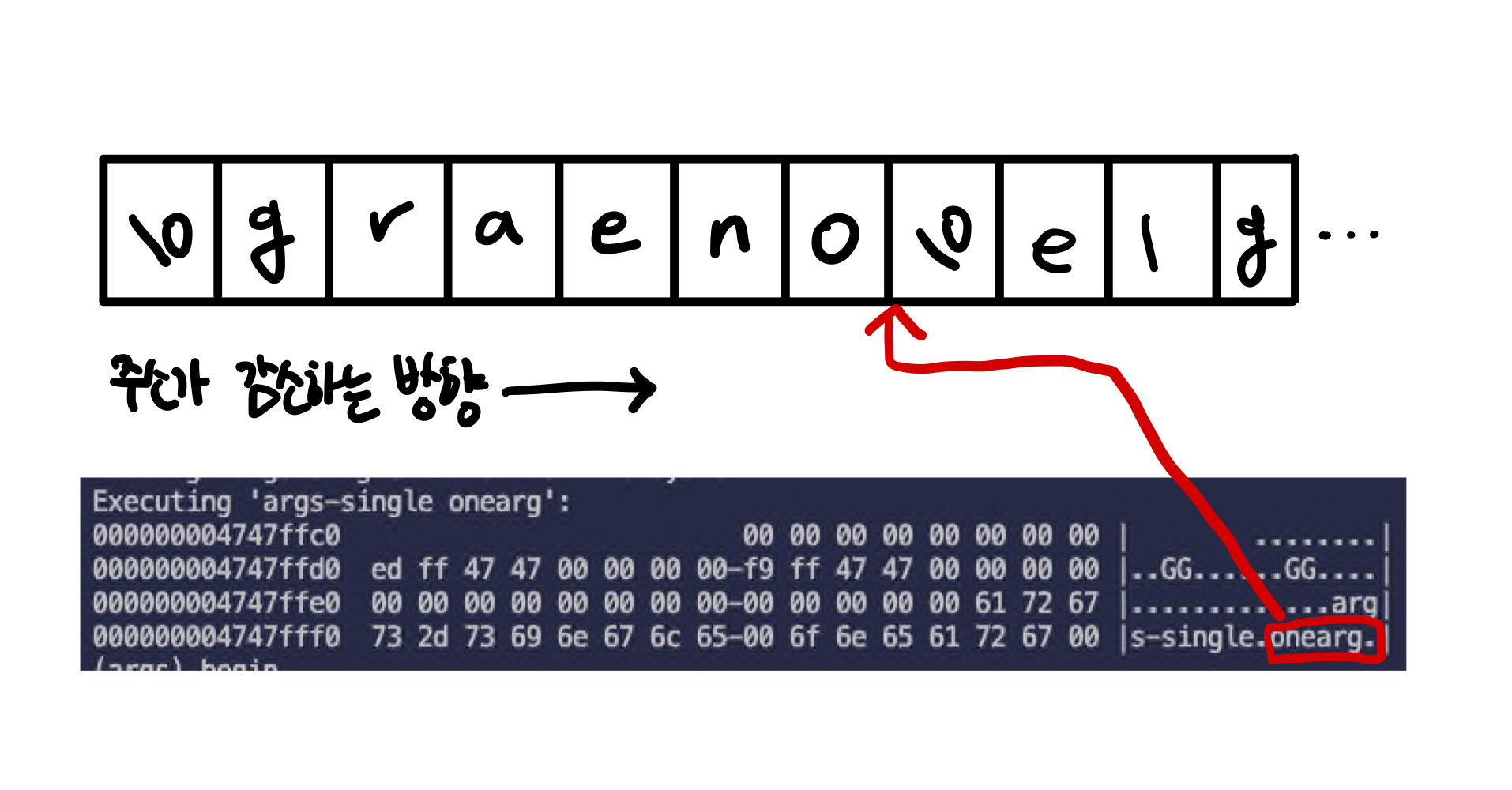
The kernel always panics when I run a custom test case. So conceptually, if we can make the changes in the original copies only when it is passed as reference so it satisfies the same.Threads/thread.c | 207 ++++++++++++++++++++. And the changes are made into the original copies of the variable. This program generates the following output:Īs you can see, in this case, the actions inside meth( ) have affected the object used as an argument. But on the higher level if we see that no other copy of the passing object is being created as in the primitive types. It may sound a bit confusing to C/C++ programmers because there is no pointers concept here and pointers directly deal with memory locations. Changes to the object inside the method affect the object used as an argument. This effectively means that objects are passed to methods by use of call-by-reference. Thus, when this reference is passed to a method, the parameter that receives it will refer to the same object as that referred to by the argument. When any object is created as below:īehind the scene, a variable of the class type is created you are creating a reference to the object. The objects themselves are never passed to a method, but the objects are always in the heap and only a reference to the object is passed to the method.
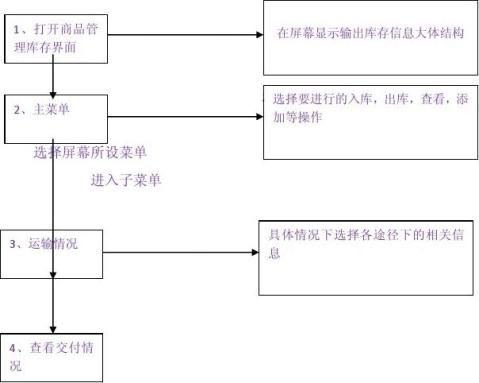
The situation changes when an object is passed to a method. The operation inside meth() did not change the on the original copy of a and b. The output from this program is shown here:Īs in the above example, the values of a and b are same before and after the calling the meth().
Pintos project2 argument passing how to#
How to Run Selenium Scripts In Tor Browser? Thus, what occurs to the parameter that receives the argument has no effect outside the method. In JAVA, when primitive types variable (int, char, float, Boolean…) are passed, they follow the pass – by – value concept. Therefore, the changes made to the passed parameter value would not affect to the original copy. In this approach the copies of the values of an argument is passed into the formal parameter of the function. Okay, let’s have a closed at both the ways with examples. The first approach is quite similar to other programming language like C/C++ but the second approach makes the JAVA different from others (C/C++). However, in Java the scenario is a bit different. Pass – by – reference or call – by – reference.Generally, in any programming language there are only two ways argument passing( parameters passing) to the function: Argument passing in java is one of the most important topic to cover, Here I am going to elaborate that what happens when different types (primitive or objects) are passed into a method in JAVA.
